Last week I posted part 1 of this series on creating plugins for Snowfakery. This second installment covers creating custom Faker Providers to use as plugins for Snowfakery.
Why create a Faker Provider instead of Snowfakery Plugin
Python’s Faker library provides many useful things, but not everything you might want. Fortunately it is designed to be extended, and Snowfakery is designed to help make it possible for those extensions to be project specific when you need to (or when it makes it easier for your team to share recipes).
Sometimes you need to have all the features of a Snowfakery plugin, like maintaining state. But often you just need to kill a gap left by the Faker community offerings. In that case, a Faker Provider may offer longer term benefits like re-usability in other projects, and useful helper functions, that give it the strong choice.
Things you’ll need
You do not need to have reviewed everything in Part 1, but you will want to look at the project structure section and to make sure you have the following tools:
- Python 3 (any recent-ish version should be fine), and the experience to read and write simple Python scripts (they aren’t any harder to follow than YML just different).
- Snowfakery 1.12 or later. Note: if you have CCI installed it contains Snowfakery but on Windows you may need extra setup.
- The Faker module for Python (probably via:
pip3 install Faker
). Not strictly required but can help you with testing. - A code editor you like that supports both Python and YML (which is pretty much anything good).
- You probably want experience working on at least one or two Snowfakery recipes.
Reminder about project structures
In part 1 we build a project around the built-in search patterns of Snowfakery.
Snowfakery looks for plugins in a select number of places:
- The Python path.
- In a
plugins
directory in the same directory as the recipe. - In a
plugins
directory below the current working directory. - A sub-directory of the user’s home directory called
.snowfakery/plugins
.
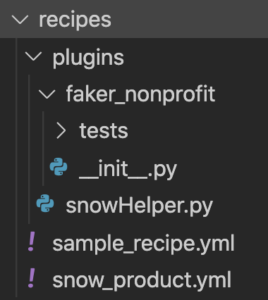
For this example the plugins directory will live within the folder with our recipe (called recipes), but you can move the plugins directory up a level and it will work just as well.
To get started, in your project create a recipes
directory. Next create a plugins
directory within recipes
. Then create faker_nonprofit
directory in plugins. You can ignore the snowHelper
and snow_product.yml
examples from part 1 in the screenshot on the right, but that’s generally what we’re doing.
Create your first Snowfakery Faker Provider
Faker itself does not have a community supported generator of Nonprofit organization names, and when you work with nonprofits a lot sometimes you need those. That is exactly what we’ll create in just a minute.
In the plugins directory you created in part 1, create a directory for your new provider with the pattern faker_[my_service_name], in my case faker_nonprofit
. Then in that new directory create a file named __init__.py
this defines the Faker provider Python module.
You can see the full working example here but I’ll walk through the outline.
The file opens by providing a doc string and importing the faker.providers module.
"""Provider for Faker which adds fake nonprofit names, and program names."""
import faker.providers
The next several lines of the faker_nonprofit
module provide arrays of words to use in the generation of fake names. Depending on what your provider does this may or may not be useful to you (but it’s very common).
Then we define the Provider itself as a class that extends the BaseProviders
class, with whatever methods you want to call:
class Provider(faker.providers.BaseProvider):
"""Provider for Faker which adds fake nonprofit information."""
def nonprofit_name(self):
"""Fake nonprofit names."""
prefix = self.random_element(PREFIXES)
suffix = self.random_element(SUFFIXES)
topic = self.random_element(TOPICS)
return " ".join([prefix, topic, suffix]).strip()
Those random element selections are from the arrays of words I mentioned a minute ago. Basically we’re just building a name from some selected words.
A note about tests
Unlike Snowfakery plugins, Faker projects have existing patterns for how to setup tests (they are not totally consistent but there are patterns out there). So the complete code on Github includes a testing module as well, and you should consider something similar for yours (particularly if you are considering making it available to the wider community). It’ll allow you to test your provider generically not just when it’s running through Snowfakery.
Linking your Provider to a Snowfakery Recipe
Now that we have a local Faker Provider, we need to connect it to our recipe.
A very simple recipe (in our recipe directory) should demonstrate the output quite nicely:
- plugin: faker_nonprofit.Provider
- object: Account
fields:
Name:
fake: nonprofit_name
Run the recipe through Snowfakery and you can see the name appears believable:
$ snowfakery recipes/sample_recipe.yml
Account(id=1, Name=Southern Unity Community)
The project uses a slightly larger recipe here that uses both plugins and generates more than one object. The full recipe in the repo will create one Salesforce Account object that has a Fake Nonprofit name, a custom field for a Main Service that will be one of our snowy puns, an address, and two related contacts with all their basic information included.
$ snowfakery recipes/sample_recipe.yml
Account(id=1, Name=Upper Friends Committee, Main_Service__c=Snowmanage, BillingStreet=4773 Giles Plains Suite 878, BillingCity=South Daniel, BillingState=Virginia, BillingPostalCode=34516, BillingCountry=United States, ShippingStreet=7844 Hester Shore Apt. 299, ShippingCity=Maynardview, ShippingState=Indiana, ShippingPostalCode=86323, ShippingCountry=United States, Phone=956.673.3002x471, Fax=+1-786-744-2112x36239, RecordType=Organization)
Contact(id=1, AccountId=Account(1), Salutation=Misc., FirstName=Isaac, LastName=Barr, Email=joypeters@example.com, Phone=+1-808-508-0989x418, MobilePhone=(987)475-7200x8072, Title=Tour manager, Birthdate=1982-03-27)
Contact(id=2, AccountId=Account(1), Salutation=Mx., FirstName=Erica, LastName=Lopez, Email=angel01@example.org, Phone=(011)243-1677x868, MobilePhone=(079)466-5474x52399, Title=Research officer, political party, Birthdate=2000-07-07)
If you have interest in seeing the Nonprofit Provider made into a more complete tool and released as its own project please let me know.
One thought on “Snowfakery Custom Plugins Part 2”